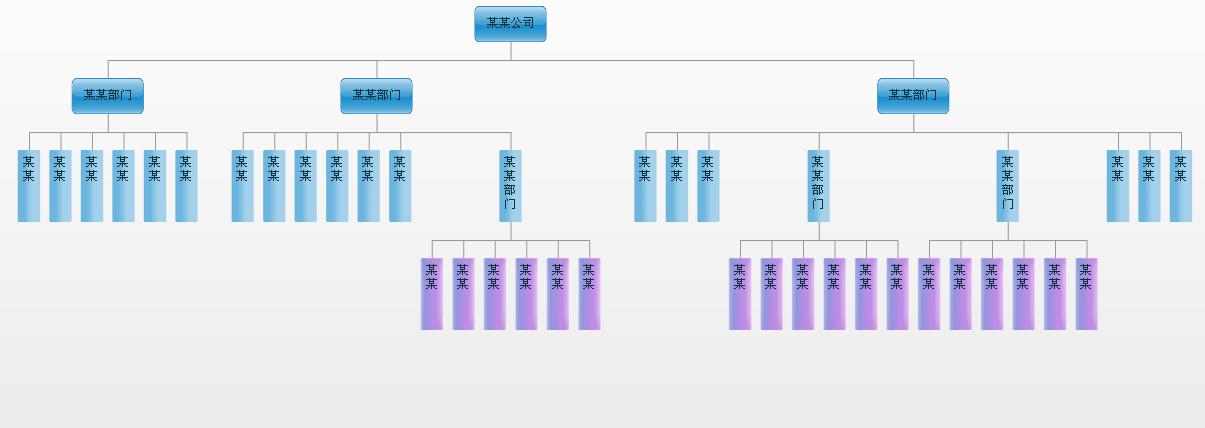
Java代码

- /**
- * create nodes,
- * calculate depth.
- * */
- private function _createSubNodes(data:ICollectionView, parentNode:IOrgChartNode):void{
- for(var cursor:IViewCursor = data.createCursor(); !cursor.afterLast; cursor.moveNext()){
- var node:IOrgChartNode = _createNode(cursor.current, parentNode);
- if(_treeDataDesciptor.isBranch(cursor.current, data)
- && _treeDataDesciptor.getChildren(cursor.current, data).length != 0){
- var __tmp:ICollectionView = _treeDataDesciptor.getChildren(cursor.current, data);
- _createSubNodes(__tmp, node);
- }
- }
- }
- private var _maxX:Number=0;
- /**
- * Wrap data with IOrgChartNode
- * */
- private function _createNode(data:Object, parentNode:IOrgChartNode):IOrgChartNode{
- var node:IOrgChartNode = new DefaultOrgChartNode();
- node.addEventListener(MouseEvent.CLICK, nodeClick);
- node.addEventListener(MouseEvent.MOUSE_OVER, nodeMouseOver);
- node.addEventListener(MouseEvent.ROLL_OVER, nodeRollOver);
- node.addEventListener(MouseEvent.MOUSE_OUT, nodeMouseOut);
- node.parentNode = parentNode;
- node.data = data;
- node.width = hItemWidth;
- node.height = hItemHeight;
- //起始时,根节点在最左边
- if(parentNode == null){
- node.x = 0;
- node.y = 0;
- //_maxX = node.width + _horizonalSpacing;
- }else{
- if(node.previousSibling == null){
- //与父节点在同一中轴线上
- //trace(node);
- node.x = parentNode.x + (parentNode.width - node.width)/2;
- _maxX = node.x + node.width;
- }else{
- node.x = _maxX + _horizonalSpacing;
- _maxX = node.x + node.width;
- }
- //移动父节点
- updateParentNodePosition(node.parentNode );
- node.y = parentNode.y + parentNode.height + _verticalSpacing;
- }
- _nodes.addItem(node);
- return node;
- }
- /**
- * 递归移动所有父节点的位置。
- * */
- private function updateParentNodePosition(node:IOrgChartNode):void{
- if(node != null){
- var subs:ArrayCollection = node.subNodes;
- var lastChild:IOrgChartNode = subs.getItemAt(subs.length - 1 ) as IOrgChartNode;
- var firstChild:IOrgChartNode = subs.getItemAt(0) as IOrgChartNode;
- node.x = firstChild.x + ( lastChild.x - firstChild.x + lastChild.width - node.width) / 2;
- //递归更新直到根节点
- updateParentNodePosition(node.parentNode);
- }
- }